Have you experience something that when you access a website it will always try to use the HTTPS scheme. This can happen because of the following reason:
The server requests a redirect to an https scheme.
Or
The browser receives an Strict-Transport-Security (STS) header .
In our case, I am talking about the second one. With the advent of Let's Encrypt CA, everybody now can have a free SSL certificate if we like that normally lasts for 3-months. To make it longer than that, just use or create a script that will do the automatic renewal of our certificate.
The server can tell the browser to always use HTTPS for a period of time using HSTS.
HTTP Strict Transport Security (HSTS) is a web security policy mechanism which helps to protect websites against protocol downgrade attacks and cookie hijacking. - https://en.wikipedia.org/wiki/HTTP_Strict_Transport_Security
We can observe HSTS in action using chrome via the developer tools. If you access a website via HTTP and we can notice from the picture at the bottom that there's an internal redirect going-on (i.e. 307 Internal Redirect). This is not a redirect request coming from the server but from the browser itself. Another clue is the existence of Non-Authoritative-Reason : HSTS header.
The Upgrade-Insecure-Request header is telling the server that we prefer the secured content to be served. Thus if our websites contains a mixture of HTTP and HTTPS artifacts (e.g. CSS, Javascript), everything will be served as HTTPS.
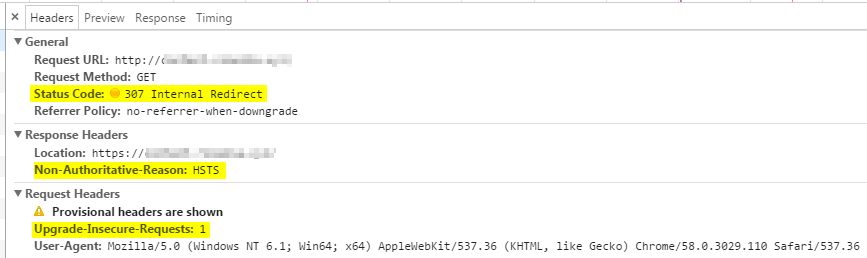
Related Post
CLEARING DOMAIN HSTS IN CHROME
Recent Comments